목차
Naive Bayes classifier
A supervised classification technique that is based on Bayes' Theorem with an assumption of independence among predictors.
나이브 베이즈 알고리즘은 베이즈 정리에 기반한다. 베이즈 정리는 각각의 사건이 일어날 확률을 측정하는 것인데, 아래의 formula를 보자.
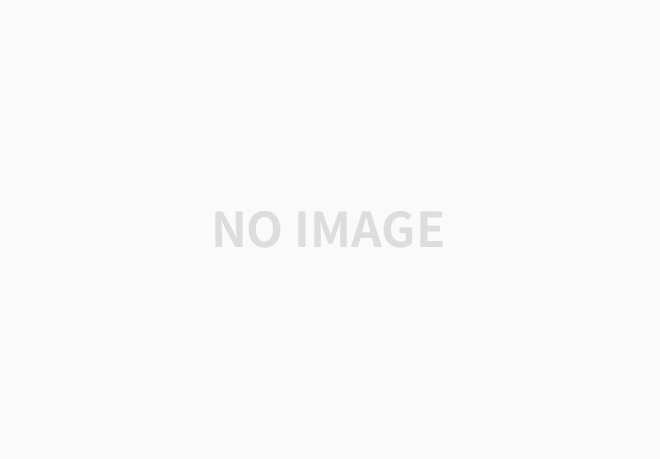
c사건과 x사건이 있다. x가 발생한 이후에 c가 발생한다고 가정하자.
P(c|x): x사건이 일어난 후 c사건이 일어날 확률이다. = 조건부 확률
P(c) : 사건 C가 일어날 확률
P(x) : 사건 x가 일어날 확률= 사건 c가 일어나기 전 사건 X가 일어날 확률 =사전확률= Predictor prior probability
P(x|c) : 사건 c가 일어났을 때, 사건 x가 앞서 일어날 확률 = 사후확률= likelihood of a predictor X given a class c
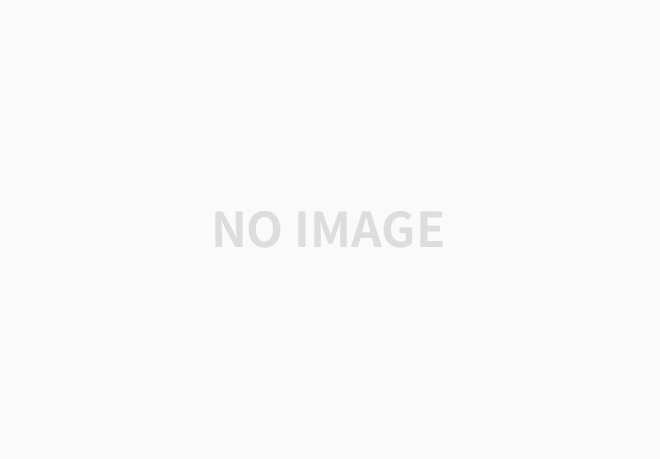
굉장히 복잡해보인다.. 사실 완벽히 이해는 되지 못했다. 다만 확실한 것은, 나이즈 베이 분류는 복잡하게 섞여 있는 문제를 비슷한 성격을 가진 특성으로 분류해낸다는 것이다.
ex) 넷플릭스 영화 추천, 콘텐츠 추천, 이메일 스팸 분류, 질병진단 등
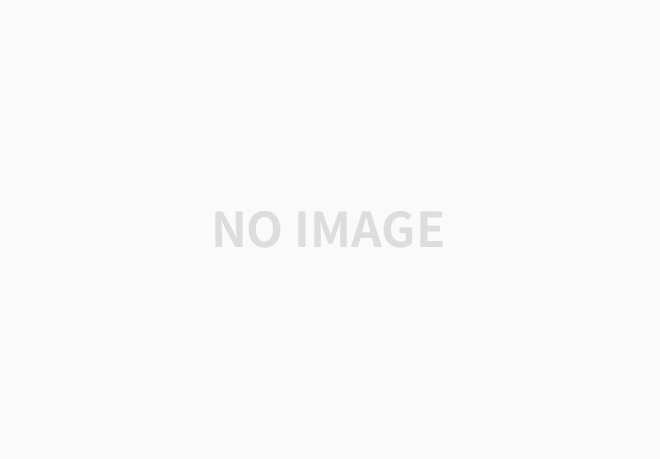
Python
1. data
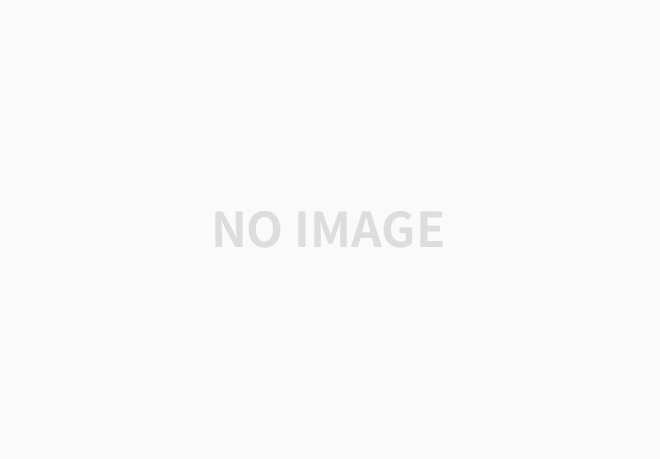
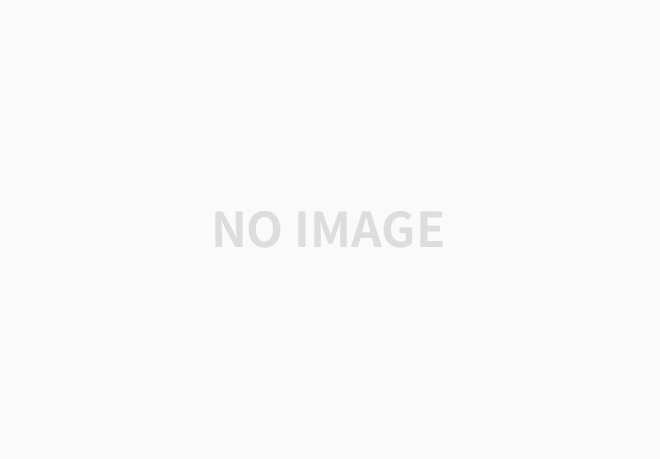
2. 나이브베이즈 이론
데이터는 완벽하지 않아서 때로는 모델의 가정을 깨뜨리기도 한다. 가능한 최선의 나이브 베이즈 모델을 만들자. 이를 위해 각각의 변수를 그 종류와 분포에 따라 처리한 후, 각각의 조건부 확률을 결합하여 최종 예측을 얻어내면 된다. 내가 손으로 일일이 계산하는 것이 아니라 파이썬을 이용해서 말이다.
사이킷런에는 나이브베이즈 알고리즘의 여러 가지 구현이 있다.
- 연속형변수: 가우시안
- 이진형: 베르누이
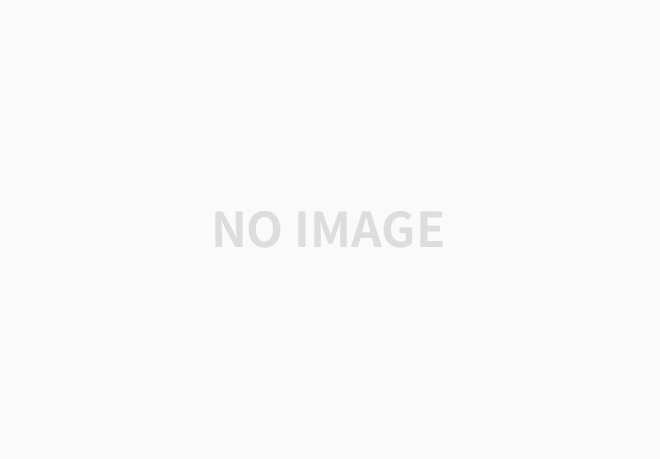
오늘은 나이브베이즈 이론이 접목된 가우시안 NB 분류기를 사용한다. 모든 변수가 연속적이고, 정규분포를 갖는다고 가정한다. 가우시안 모델은 불완전한 데이터라도 꽤 괜찮은 결과를 산출한다.
3. 라이브러리
import numpy as np
import pandas as pd
import matplotlib.pyplot as plt
from sklearn.model_selection import train_test_split
from sklearn.naive_bayes import GaussianNB
from sklearn.metrics import recall_score, precision_score, f1_score, accuracy_score
from sklearn.metrics import confusion_matrix, ConfusionMatrixDisplay
4. 데이터 밸런스 체크
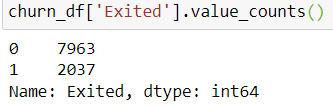
5. feature selection
churn_df = churn_df.drop(['Tenure', 'Age'], axis=1)
6. 데이터 분할
★ stratify
training, test 데이터에 y 변수에서 발견된 클래스 비율을 사용해야 한다는 의미이다. 즉, 실제 데이터와 비슷하게 계층화하는 것이다. 예를 들어 stratify를 정하지 않으면 random하게 분리된 데이터셋에서 클래스가 모두0인 것만 있을 수도 있다. 따라서 stratify할 필요가 있다.
# Define the y (target) variable
y = churn_df['Exited']
# Define the X (predictor) variables
X = churn_df.copy()
X = X.drop('Exited', axis=1)
# Split into train and test sets
X_train, X_test, y_train, y_test = train_test_split(X, y, test_size=0.25, \
stratify=y, random_state=42)
7. 모델링
# Fit the model
gnb = GaussianNB()
gnb.fit(X_train, y_train)
# Get the predictions on test data
y_preds = gnb.predict(X_test)
8. 평가
print('Accuracy:', '%.3f' % accuracy_score(y_test, y_preds))
print('Precision:', '%.3f' % precision_score(y_test, y_preds))
print('Recall:', '%.3f' % recall_score(y_test, y_preds))
print('F1 Score:', '%.3f' % f1_score(y_test, y_preds))
Accuracy: 0.796
Precision: 0.000
Recall: 0.000
F1 Score: 0.000
----> 문제가 있음을 인지한다.
9. 모델 확인
# 예상값
np.unique(y_preds)
-->0 밖에 없다.
10. 데이터 확인
X.describe()
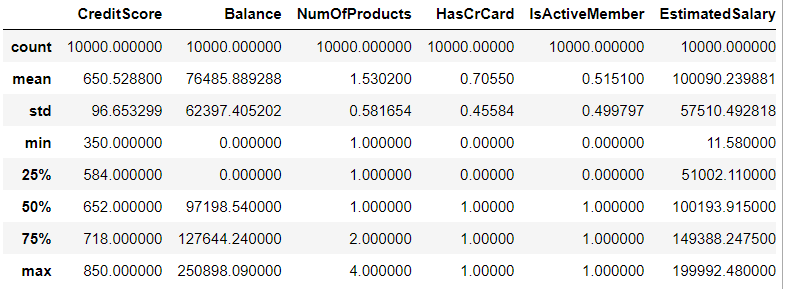
데이터가 스케일링 되지 않았다.
11. 스케일링
MinMaxScaler를 이용한다.
# Import the scaler function
from sklearn.preprocessing import MinMaxScaler
# Instantiate the scaler
scaler = MinMaxScaler()
# Fit the scaler to the training data
scaler.fit(X_train)
# Scale the training data
X_train = scaler.transform(X_train)
# Scale the test data
X_test = scaler.transform(X_test)
12. 스케일된 데이터로 모델 만들기
# Fit the model
gnb_scaled = GaussianNB()
gnb_scaled.fit(X_train, y_train)
# Get the predictions on test data
scaled_preds = gnb_scaled.predict(X_test)
np.unique(scaled_preds)
-->
array([0, 1])
13. 모델 평가
print('Accuracy:', '%.3f' % accuracy_score(y_test, scaled_preds))
print('Precision:', '%.3f' % precision_score(y_test,scaled_preds))
print('Recall:', '%.3f' % recall_score(y_test, scaled_preds))
print('F1 Score:', '%.3f' % f1_score(y_test, scaled_preds))
14. confusion 매트릭스
def conf_matrix_plot(model, x_data, y_data):
'''
Accepts as argument model object, X data (test or validate), and y data (test or validate).
Return a plot of confusion matrix for predictions on y data.
'''
model_pred = model.predict(x_data)
cm = confusion_matrix(y_data, model_pred, labels=model.classes_)
disp = ConfusionMatrixDisplay(confusion_matrix=cm,
display_labels=model.classes_,
)
disp.plot(values_format='') # `values_format=''` suppresses scientific notation
plt.show()
conf_matrix_plot(gnb_scaled, X_test, y_test)
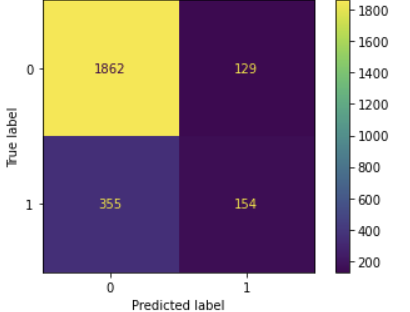